개요
이번에 새로운 프로젝트를 시작하면서 hasura를 사용해 보자는 제안이 있었다.
기존에 hasura를 사용 해 본 적은 있긴 하였으나 cloud 형태로 사용해 보았기 때문에 로컬 서버의 구축과 배포등을 어떻게 해야 할 지 고민이 되었다.
일단 데이터베이스 마이그레이션과 시딩을 해 줘야 하는데 hasura에서 제공해 주긴 하지만, factory 관리와 모델 관리등 조금 더 프로그래밍 적으로 관리하고 싶은 마음이 생겼다.
그렇기 때문에 기존에 자주 사용하던 laravel과 연동하여서 시스템을 구축해 보았다.
현재 생각하는 바로는 laravel로 관리할 대상 포인트 들은 다음과 같다.
- 데이터 모델
- 데이터베이스 마이그레이션
- 데이터베이스 시딩
- 인증
- 파일 업로드 관리
구축 준비
먼저, php 에서 postgresql이 연동 되게끔 처리가 안 되어있으면 오류가 발생하니, window 환경이라면 php.ini 파일에서 pgsql, pdo-pgsql 앞에 주석을 해제 해 주도록 하자.
ubuntu 환경으로는 ext 파일을 다운 받아 준다.
sudo apt-get install php-pgsql
이 외에 기본적으로 구성 되어 있어야 할 것들은 다음과 같다.
- docker
- composer
구축
1. Laravel install
$ composer create-project laravel/laravel hasura-graphql
2. sail 설치
$ cd hasura-graphql
$ composer require laravel/sail --dev
3. sail install, pgsql 선택
$ php artisan sail:install
4. docker-compose.yml
파일에 hasura engine 추가
graphql-engine:
image: hasura/graphql-engine:v2.35.0
ports:
- '8080:8080'
restart: always
environment:
## postgres database to store Hasura metadata
HASURA_GRAPHQL_METADATA_DATABASE_URL: postgres://${DB_USERNAME}:${DB_PASSWORD}@pgsql:${FORWARD_DB_PORT:-5432}/${DB_DATABASE}
HASURA_GRAPHQL_DATABASE_URL: postgres://${DB_USERNAME}:${DB_PASSWORD}@pgsql:${FORWARD_DB_PORT:-5432}/${DB_DATABASE}
## enable the console served by server
HASURA_GRAPHQL_ENABLE_CONSOLE: 'true' # set to "false" to disable console
## enable debugging mode. It is recommended to disable this in production
HASURA_GRAPHQL_DEV_MODE: 'true'
HASURA_GRAPHQL_ENABLED_LOG_TYPES: startup, http-log, webhook-log, websocket-log, query-log
## uncomment next line to set an admin secret
# HASURA_GRAPHQL_ADMIN_SECRET: ${HASURA_GRAPHQL_ADMIN_SECRET}
# HASURA_GRAPHQL_UNAUTHORIZED_ROLE: public
volumes:
- './hasura/metadata:/hasura-metadata'
networks:
- sail
depends_on:
- pgsql
5. 환경변수 등록 (넘어가도 됩니다. 넘어갈 경우, ./vendor/bin/sail 로 sail 실행)
$ alias sail='[ -f sail ] && sh sail || sh vendor/bin/sail'
$ sail up
추가적으로, 80번 포트가 기존에 사용중 이어서 .env에 다음과 APP_PORT를 추가해 주었다.
bashAPP_PORT=81
81번 포트에서 다음과 같이 Laravel 기본 index 화면이 노출 된다.
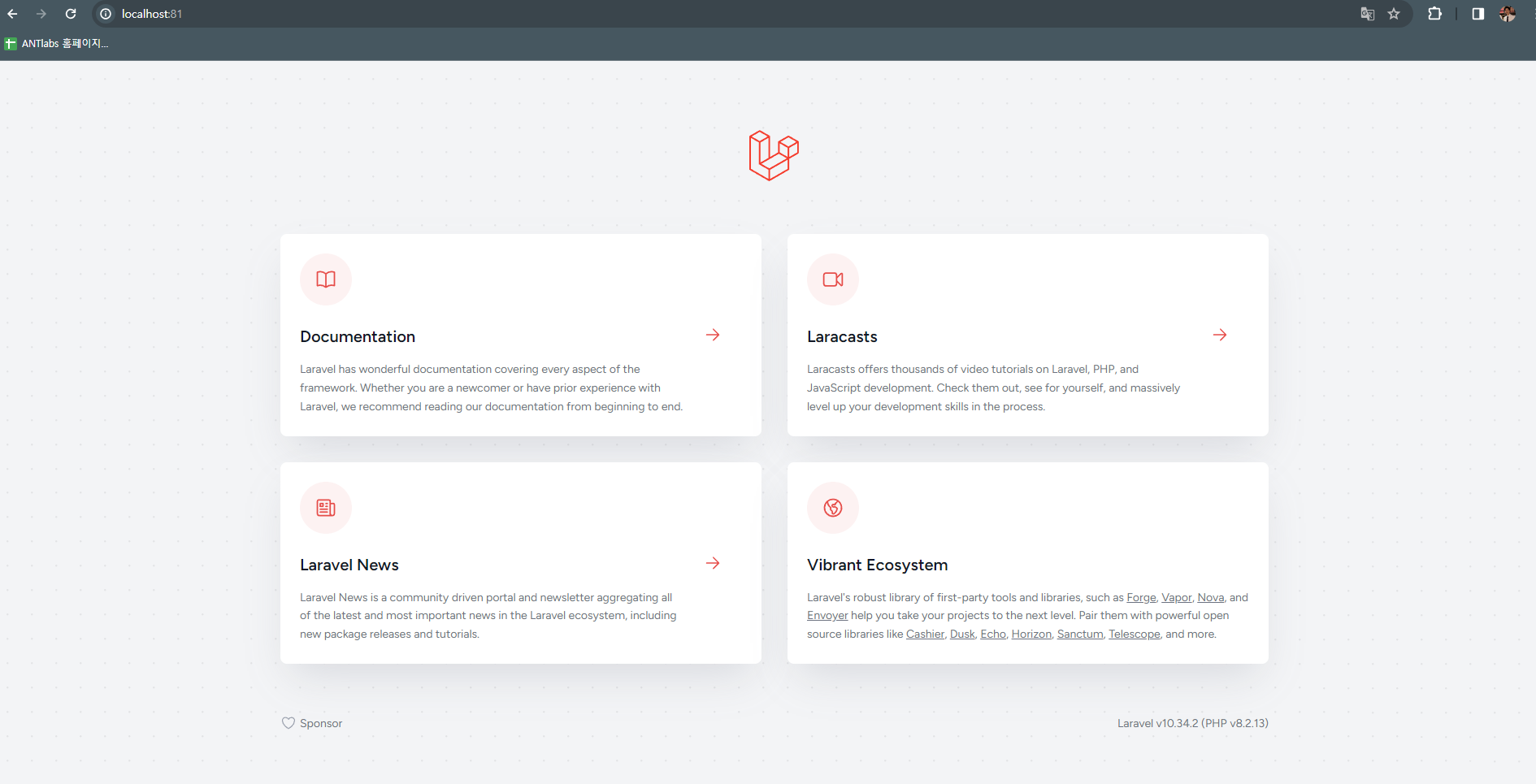
8080포트로 진입하게 되면 hasura의 index가 노출된다.
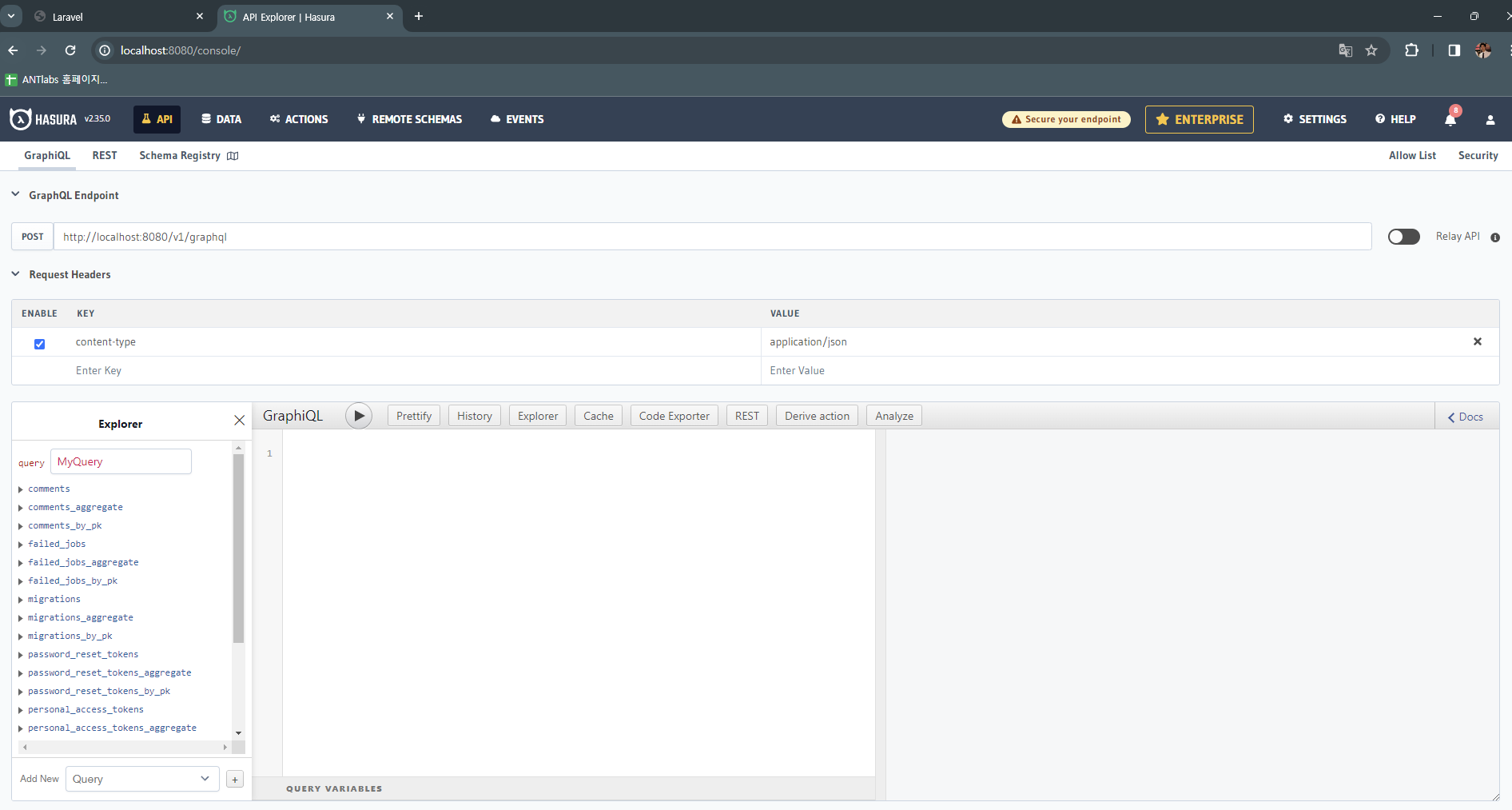
기본 테스트
1. 간단하게 두개의 모델을 정의하며, seed를 위한 factory와 migration 파일 생성
$ php artisan make:model Post --factory --migration
$ php artisan make:model Comment --factory --migration
2. migrations create table
코드
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
$table->softDeletes();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('posts');
}
};
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->text('content');
$table->timestamps();
$table->softDeletes();
$table->foreignid('post_id')
->constrained()
->onDelete('cascade');
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('comments');
}
};
3. model
코드
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
use HasFactory, SoftDeletes;
public function comments() {
return $this->hasMany(Comment::class);
}
}
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Comment extends Model
{
use HasFactory, SoftDeletes;
}
4. factory
코드
<?php
namespace Database\Factories;
use Illuminate\Database\Eloquent\Factories\Factory;
/**
* @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Post>
*/
class PostFactory extends Factory
{
/**
* Define the model's default state.
*
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'title'=>$this->faker->sentence(),
'content'=>$this->faker->sentence()
];
}
}
<?php
namespace Database\Factories;
use App\Models\Post;
use Illuminate\Database\Eloquent\Factories\Factory;
/**
* @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Comment>
*/
class CommentFactory extends Factory
{
/**
* Define the model's default state.
*
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'post_id'=>Post::factory(),
'content'=>$this->faker->sentence()
];
}
}
5. DatabaseSeeder.php
코드
<?php
namespace Database\Seeders;
// use Illuminate\Database\Console\Seeds\WithoutModelEvents;
use App\Models\Post;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*/
public function run(): void
{
// \App\Models\User::factory(10)->create();
// \App\Models\User::factory()->create([
// 'name' => 'Test User',
// 'email' => 'test@example.com',
// ]);
Post::factory()
->hasComments(5)
->count(3)
->create();
}
}
5. 마이그레이션
bash$ php artisan migrate:fresh --seed
시딩과 같이 마이그레이션을 처리해 준다.
먼저, 값이 잘 들어갔는지 확인 해 본다.
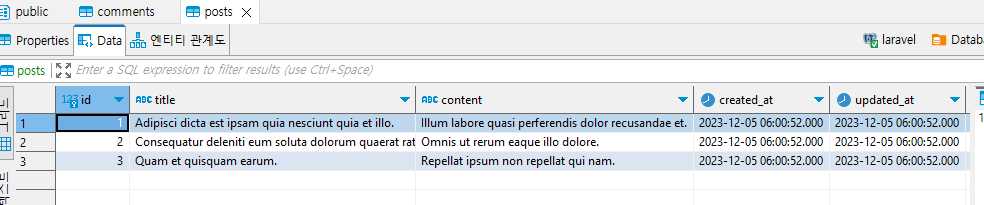
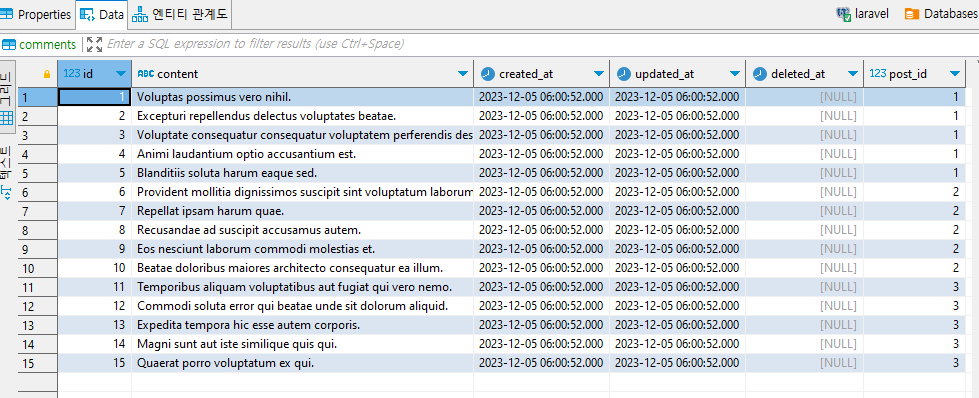
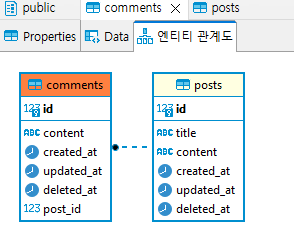
6. hasura Tracking
마이그레이션 이후, hasura의 DATA 부분에 들어가면 Untracked tables or view에 무언가가 추가 되어 있다.
Track All을 눌러서 hasura에서 track을 해 주자.
만약 DB 명이 다르게 설정 되어 있다면, Edit Postgres Connection 에서 Database name을 env에 설정한 값으로 변경 해 주면 된다.
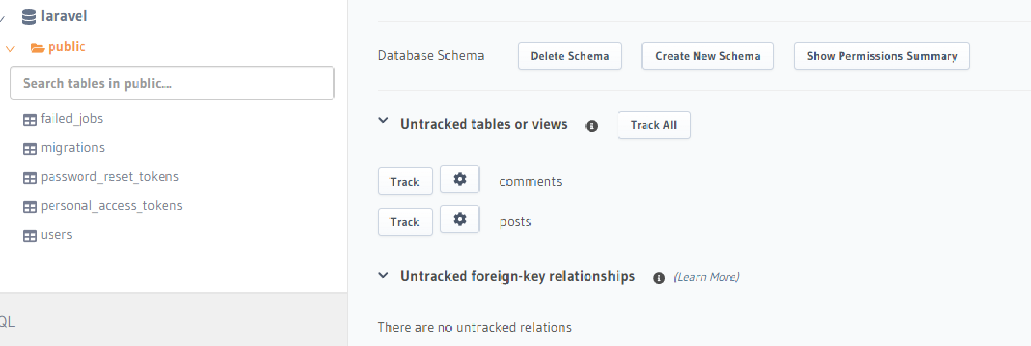
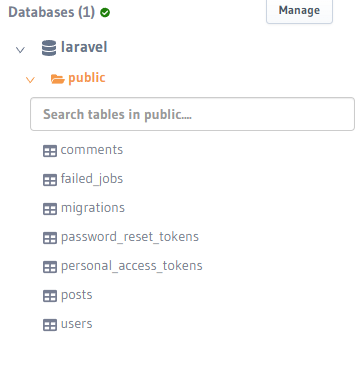
7. graphql Test
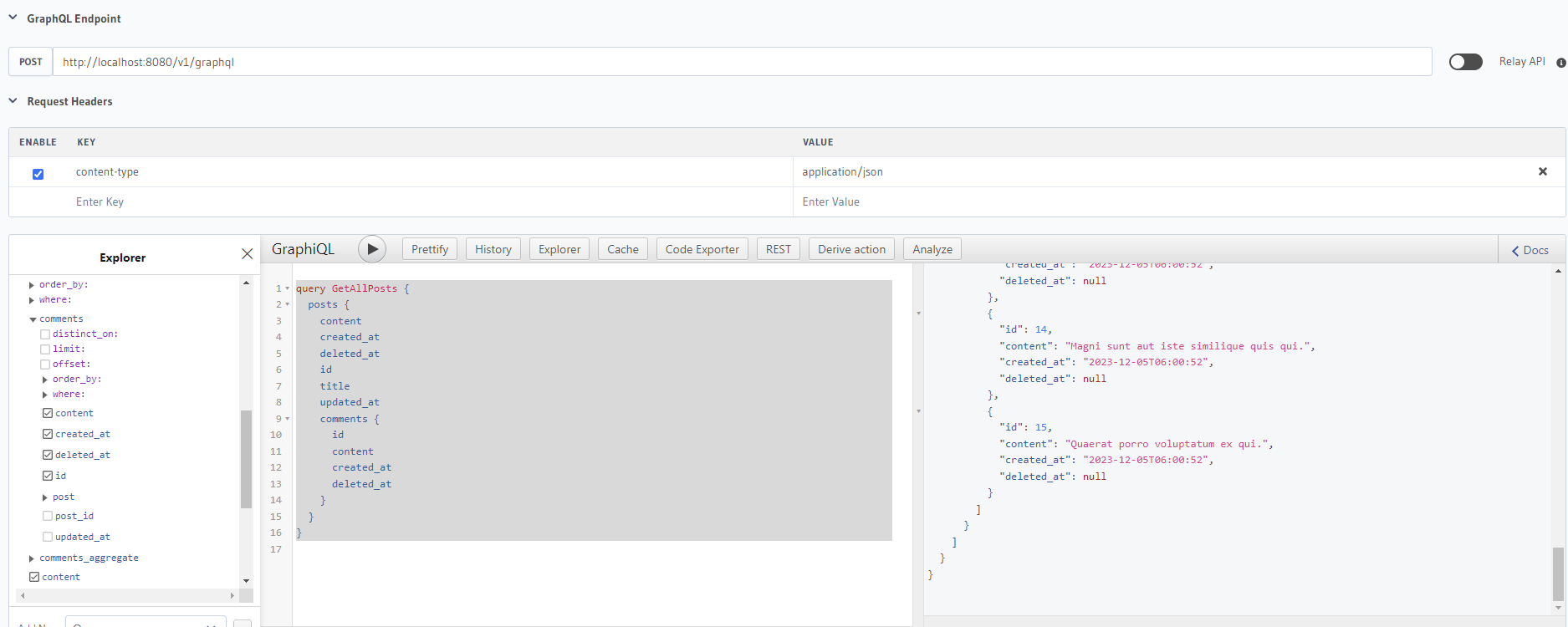
아래와 같이 쿼리를 작성하고 실행 해 보면 정상적으로 값이 오는 것을 확인 할 수 있다.
bashquery GetAllPosts {
posts {
content
created_at
deleted_at
id
title
updated_at
comments {
id
content
created_at
deleted_at
}
}
}
bash{
"data": {
"posts": [
{
"content": "Illum labore quasi perferendis dolor recusandae et.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 1,
"title": "Adipisci dicta est ipsam quia nesciunt quia et illo.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 1,
"content": "Voluptas possimus vero nihil.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 2,
"content": "Excepturi repellendus delectus voluptates beatae.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 3,
"content": "Voluptate consequatur consequatur voluptatem perferendis deserunt.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 4,
"content": "Animi laudantium optio accusantium est.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 5,
"content": "Blanditiis soluta harum eaque sed.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
},
{
"content": "Omnis ut rerum eaque illo dolore.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 2,
"title": "Consequatur deleniti eum soluta dolorum quaerat ratione sunt.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 6,
"content": "Provident mollitia dignissimos suscipit sint voluptatum laborum vitae dolorem.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 7,
"content": "Repellat ipsam harum quae.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 8,
"content": "Recusandae ad suscipit accusamus autem.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 9,
"content": "Eos nesciunt laborum commodi molestias et.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 10,
"content": "Beatae doloribus maiores architecto consequatur ea illum.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
},
{
"content": "Repellat ipsum non repellat qui nam.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 3,
"title": "Quam et quisquam earum.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 11,
"content": "Temporibus aliquam voluptatibus aut fugiat qui vero nemo.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 12,
"content": "Commodi soluta error qui beatae unde sit dolorum aliquid.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 13,
"content": "Expedita tempora hic esse autem corporis.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 14,
"content": "Magni sunt aut iste similique quis qui.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 15,
"content": "Quaerat porro voluptatum ex qui.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
}
]
}
}
참조
https://robmellett.com/posts/laravel-and-hasura-for-instant-graphql
Laravel And Hasura for Instant GraphQL
How to use Laravel and Hasura for Instant GraphQL
robmellett.com
'Programming' 카테고리의 다른 글
Laravel + Hasura +Protgresql - Environment 관리 (0) | 2023.12.06 |
---|---|
Laravel + hasura + postgresql - lighthouse, playground 설정 (0) | 2023.12.05 |
Oauth2.0 + API Server(Laravel Sanctum) + 통합인증 구현 (1) | 2023.08.30 |
[PHP Laravel] 대용량 트래픽 관련하여.. + Chat GPT (0) | 2023.04.02 |
[Next.js] localstorage is not defined (0) | 2023.03.25 |
개요
이번에 새로운 프로젝트를 시작하면서 hasura를 사용해 보자는 제안이 있었다.
기존에 hasura를 사용 해 본 적은 있긴 하였으나 cloud 형태로 사용해 보았기 때문에 로컬 서버의 구축과 배포등을 어떻게 해야 할 지 고민이 되었다.
일단 데이터베이스 마이그레이션과 시딩을 해 줘야 하는데 hasura에서 제공해 주긴 하지만, factory 관리와 모델 관리등 조금 더 프로그래밍 적으로 관리하고 싶은 마음이 생겼다.
그렇기 때문에 기존에 자주 사용하던 laravel과 연동하여서 시스템을 구축해 보았다.
현재 생각하는 바로는 laravel로 관리할 대상 포인트 들은 다음과 같다.
- 데이터 모델
- 데이터베이스 마이그레이션
- 데이터베이스 시딩
- 인증
- 파일 업로드 관리
구축 준비
먼저, php 에서 postgresql이 연동 되게끔 처리가 안 되어있으면 오류가 발생하니, window 환경이라면 php.ini 파일에서 pgsql, pdo-pgsql 앞에 주석을 해제 해 주도록 하자.
ubuntu 환경으로는 ext 파일을 다운 받아 준다.
sudo apt-get install php-pgsql
이 외에 기본적으로 구성 되어 있어야 할 것들은 다음과 같다.
- docker
- composer
구축
1. Laravel install
$ composer create-project laravel/laravel hasura-graphql
2. sail 설치
$ cd hasura-graphql
$ composer require laravel/sail --dev
3. sail install, pgsql 선택
$ php artisan sail:install
4. docker-compose.yml
파일에 hasura engine 추가
graphql-engine:
image: hasura/graphql-engine:v2.35.0
ports:
- '8080:8080'
restart: always
environment:
## postgres database to store Hasura metadata
HASURA_GRAPHQL_METADATA_DATABASE_URL: postgres://${DB_USERNAME}:${DB_PASSWORD}@pgsql:${FORWARD_DB_PORT:-5432}/${DB_DATABASE}
HASURA_GRAPHQL_DATABASE_URL: postgres://${DB_USERNAME}:${DB_PASSWORD}@pgsql:${FORWARD_DB_PORT:-5432}/${DB_DATABASE}
## enable the console served by server
HASURA_GRAPHQL_ENABLE_CONSOLE: 'true' # set to "false" to disable console
## enable debugging mode. It is recommended to disable this in production
HASURA_GRAPHQL_DEV_MODE: 'true'
HASURA_GRAPHQL_ENABLED_LOG_TYPES: startup, http-log, webhook-log, websocket-log, query-log
## uncomment next line to set an admin secret
# HASURA_GRAPHQL_ADMIN_SECRET: ${HASURA_GRAPHQL_ADMIN_SECRET}
# HASURA_GRAPHQL_UNAUTHORIZED_ROLE: public
volumes:
- './hasura/metadata:/hasura-metadata'
networks:
- sail
depends_on:
- pgsql
5. 환경변수 등록 (넘어가도 됩니다. 넘어갈 경우, ./vendor/bin/sail 로 sail 실행)
$ alias sail='[ -f sail ] && sh sail || sh vendor/bin/sail'
$ sail up
추가적으로, 80번 포트가 기존에 사용중 이어서 .env에 다음과 APP_PORT를 추가해 주었다.
bashAPP_PORT=81
81번 포트에서 다음과 같이 Laravel 기본 index 화면이 노출 된다.
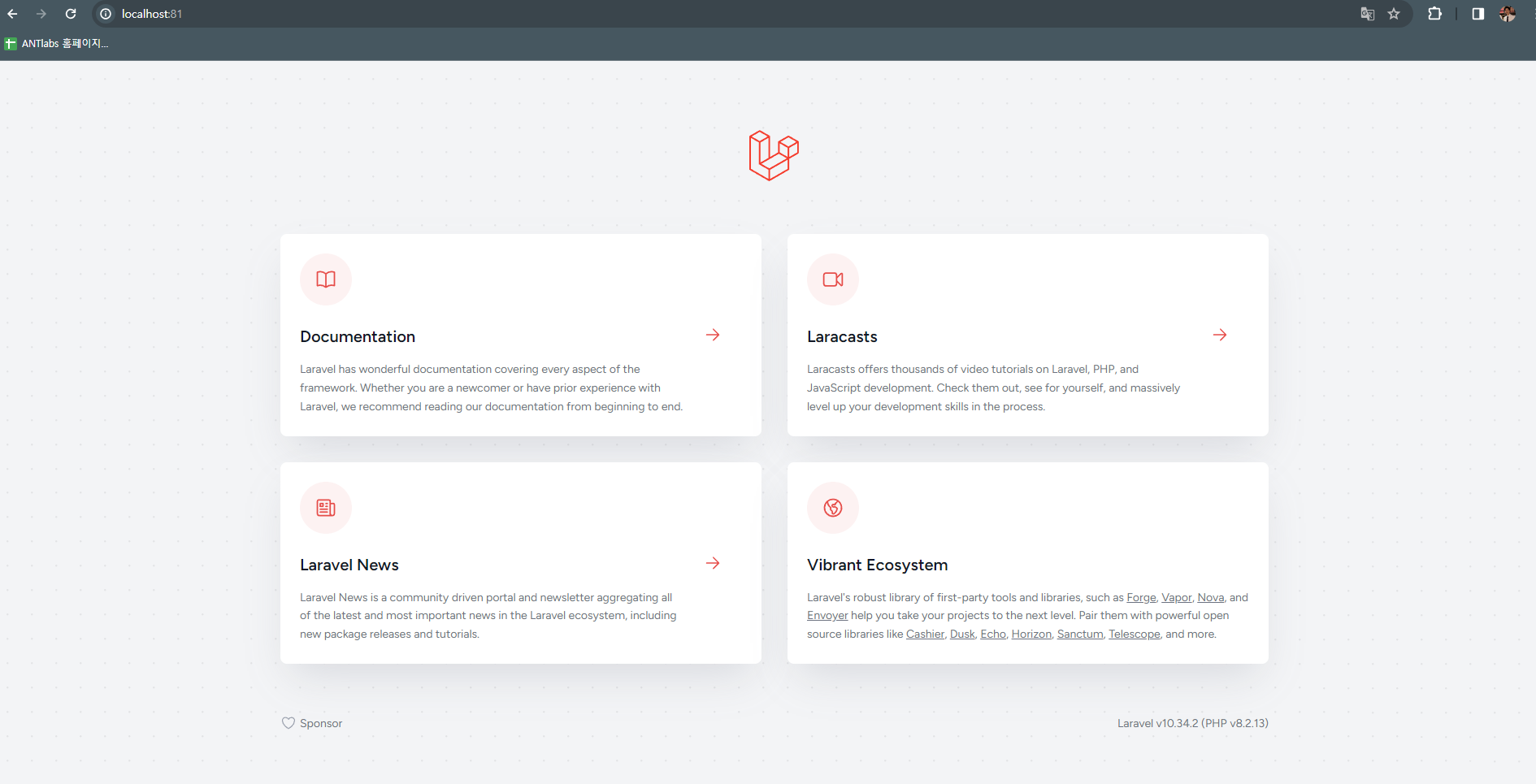
8080포트로 진입하게 되면 hasura의 index가 노출된다.
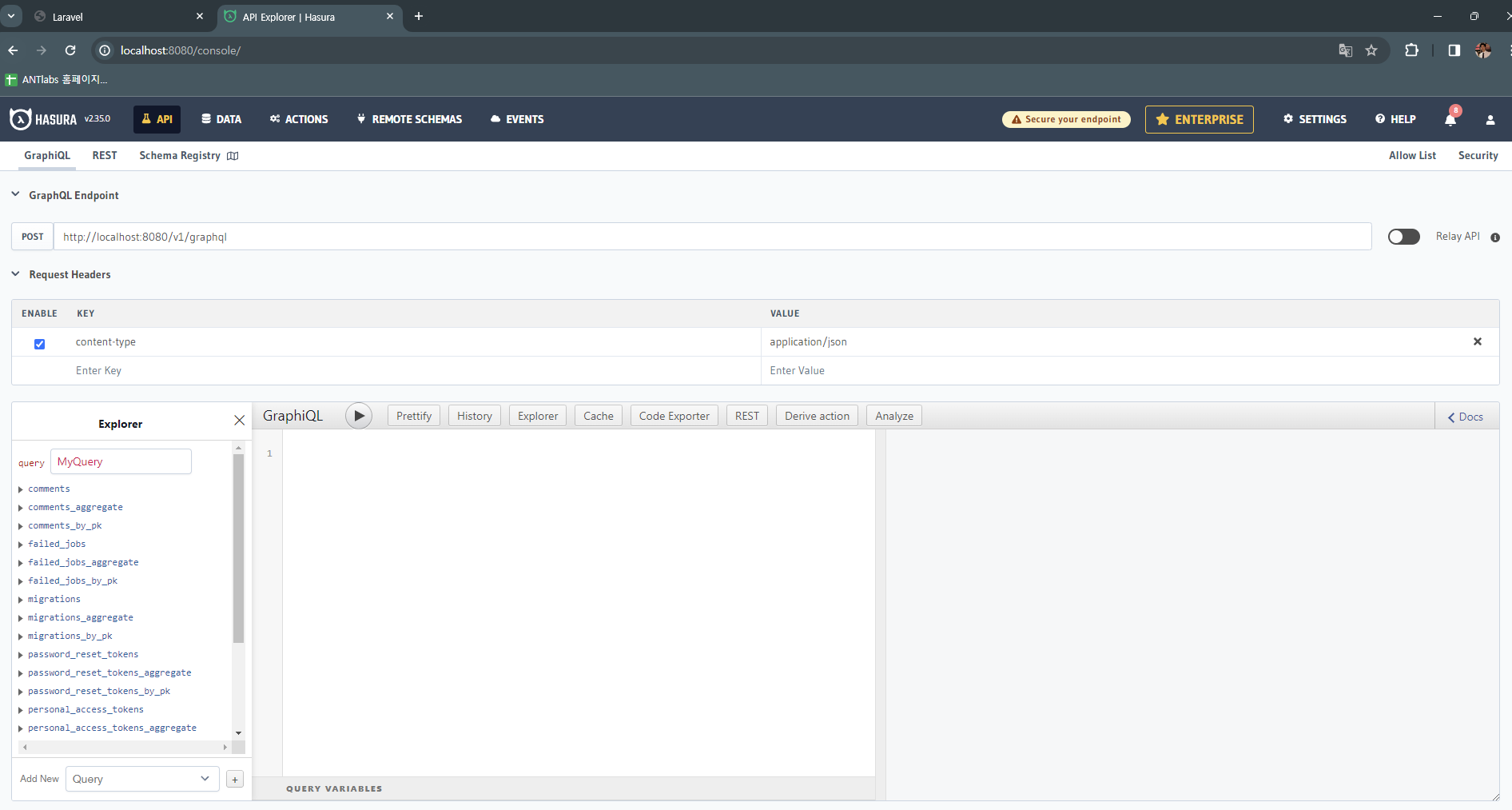
기본 테스트
1. 간단하게 두개의 모델을 정의하며, seed를 위한 factory와 migration 파일 생성
$ php artisan make:model Post --factory --migration
$ php artisan make:model Comment --factory --migration
2. migrations create table
코드
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
$table->softDeletes();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('posts');
}
};
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->text('content');
$table->timestamps();
$table->softDeletes();
$table->foreignid('post_id')
->constrained()
->onDelete('cascade');
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('comments');
}
};
3. model
코드
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Post extends Model
{
use HasFactory, SoftDeletes;
public function comments() {
return $this->hasMany(Comment::class);
}
}
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Comment extends Model
{
use HasFactory, SoftDeletes;
}
4. factory
코드
<?php
namespace Database\Factories;
use Illuminate\Database\Eloquent\Factories\Factory;
/**
* @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Post>
*/
class PostFactory extends Factory
{
/**
* Define the model's default state.
*
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'title'=>$this->faker->sentence(),
'content'=>$this->faker->sentence()
];
}
}
<?php
namespace Database\Factories;
use App\Models\Post;
use Illuminate\Database\Eloquent\Factories\Factory;
/**
* @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Comment>
*/
class CommentFactory extends Factory
{
/**
* Define the model's default state.
*
* @return array<string, mixed>
*/
public function definition(): array
{
return [
'post_id'=>Post::factory(),
'content'=>$this->faker->sentence()
];
}
}
5. DatabaseSeeder.php
코드
<?php
namespace Database\Seeders;
// use Illuminate\Database\Console\Seeds\WithoutModelEvents;
use App\Models\Post;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*/
public function run(): void
{
// \App\Models\User::factory(10)->create();
// \App\Models\User::factory()->create([
// 'name' => 'Test User',
// 'email' => 'test@example.com',
// ]);
Post::factory()
->hasComments(5)
->count(3)
->create();
}
}
5. 마이그레이션
bash$ php artisan migrate:fresh --seed
시딩과 같이 마이그레이션을 처리해 준다.
먼저, 값이 잘 들어갔는지 확인 해 본다.
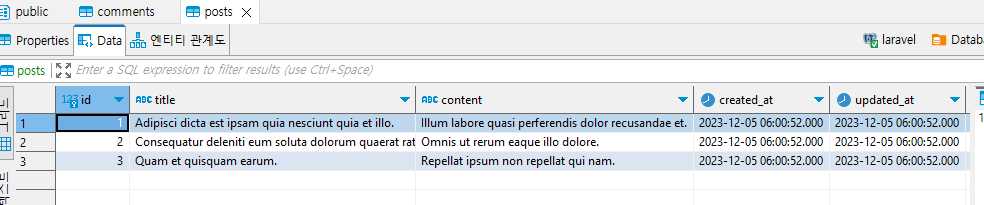
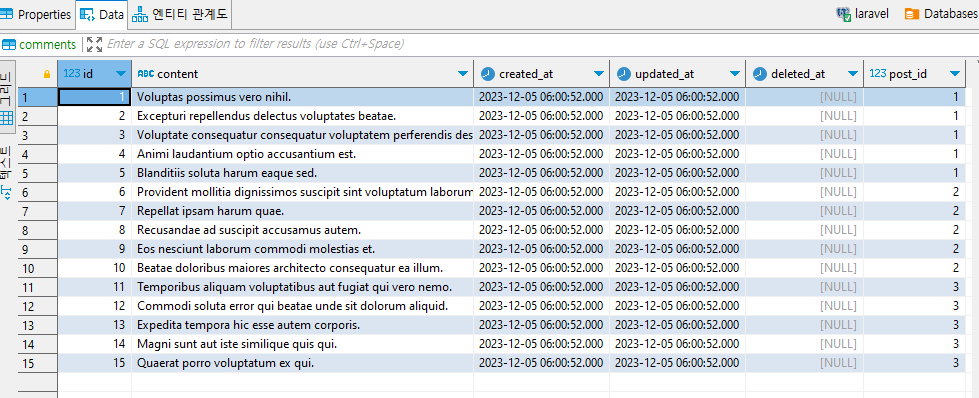
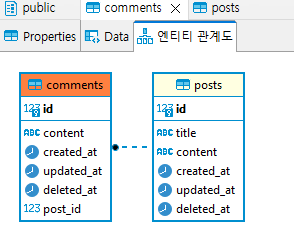
6. hasura Tracking
마이그레이션 이후, hasura의 DATA 부분에 들어가면 Untracked tables or view에 무언가가 추가 되어 있다.
Track All을 눌러서 hasura에서 track을 해 주자.
만약 DB 명이 다르게 설정 되어 있다면, Edit Postgres Connection 에서 Database name을 env에 설정한 값으로 변경 해 주면 된다.
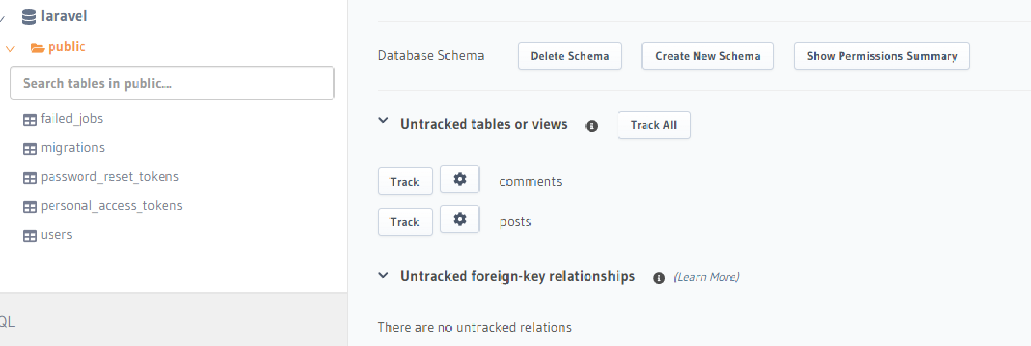
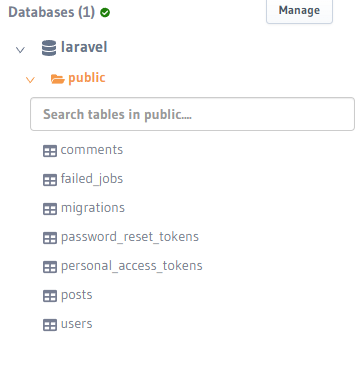
7. graphql Test
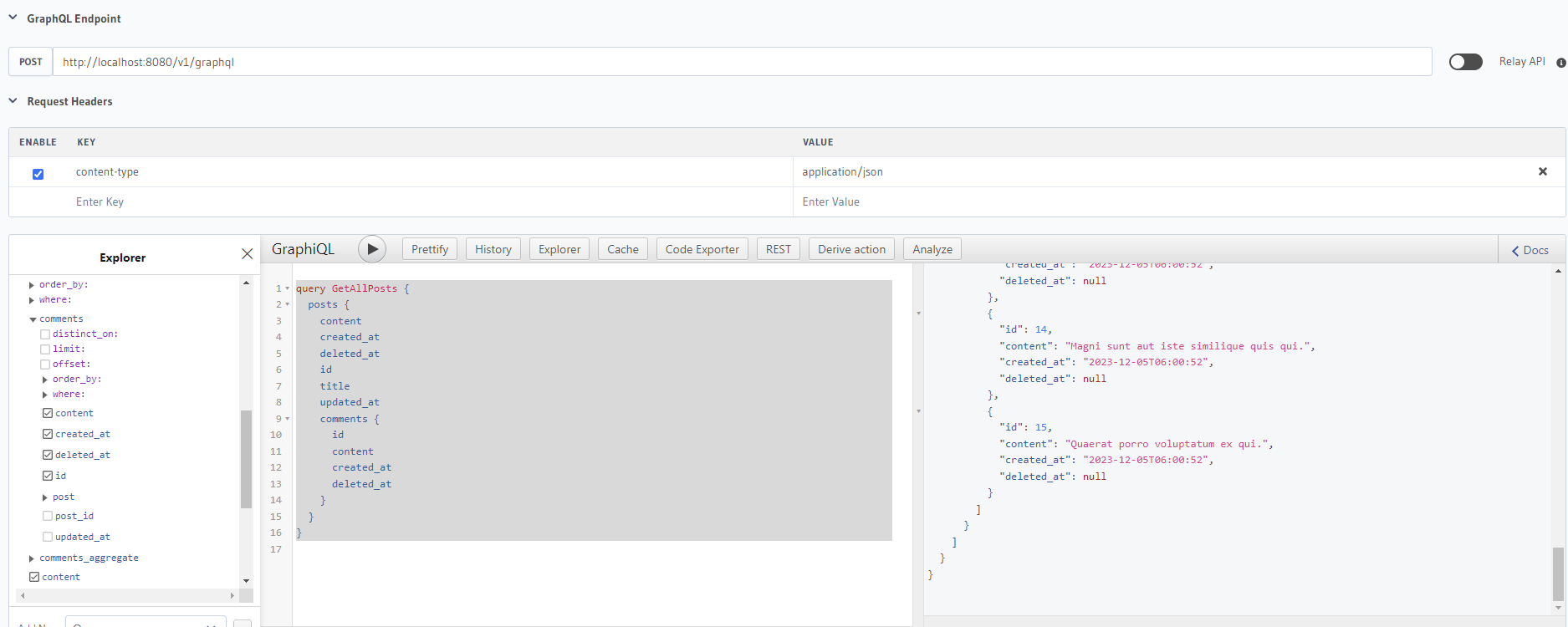
아래와 같이 쿼리를 작성하고 실행 해 보면 정상적으로 값이 오는 것을 확인 할 수 있다.
bashquery GetAllPosts {
posts {
content
created_at
deleted_at
id
title
updated_at
comments {
id
content
created_at
deleted_at
}
}
}
bash{
"data": {
"posts": [
{
"content": "Illum labore quasi perferendis dolor recusandae et.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 1,
"title": "Adipisci dicta est ipsam quia nesciunt quia et illo.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 1,
"content": "Voluptas possimus vero nihil.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 2,
"content": "Excepturi repellendus delectus voluptates beatae.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 3,
"content": "Voluptate consequatur consequatur voluptatem perferendis deserunt.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 4,
"content": "Animi laudantium optio accusantium est.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 5,
"content": "Blanditiis soluta harum eaque sed.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
},
{
"content": "Omnis ut rerum eaque illo dolore.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 2,
"title": "Consequatur deleniti eum soluta dolorum quaerat ratione sunt.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 6,
"content": "Provident mollitia dignissimos suscipit sint voluptatum laborum vitae dolorem.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 7,
"content": "Repellat ipsam harum quae.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 8,
"content": "Recusandae ad suscipit accusamus autem.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 9,
"content": "Eos nesciunt laborum commodi molestias et.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 10,
"content": "Beatae doloribus maiores architecto consequatur ea illum.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
},
{
"content": "Repellat ipsum non repellat qui nam.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null,
"id": 3,
"title": "Quam et quisquam earum.",
"updated_at": "2023-12-05T06:00:52",
"comments": [
{
"id": 11,
"content": "Temporibus aliquam voluptatibus aut fugiat qui vero nemo.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 12,
"content": "Commodi soluta error qui beatae unde sit dolorum aliquid.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 13,
"content": "Expedita tempora hic esse autem corporis.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 14,
"content": "Magni sunt aut iste similique quis qui.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
},
{
"id": 15,
"content": "Quaerat porro voluptatum ex qui.",
"created_at": "2023-12-05T06:00:52",
"deleted_at": null
}
]
}
]
}
}
참조
https://robmellett.com/posts/laravel-and-hasura-for-instant-graphql
Laravel And Hasura for Instant GraphQL
How to use Laravel and Hasura for Instant GraphQL
robmellett.com
'Programming' 카테고리의 다른 글
Laravel + Hasura +Protgresql - Environment 관리 (0) | 2023.12.06 |
---|---|
Laravel + hasura + postgresql - lighthouse, playground 설정 (0) | 2023.12.05 |
Oauth2.0 + API Server(Laravel Sanctum) + 통합인증 구현 (1) | 2023.08.30 |
[PHP Laravel] 대용량 트래픽 관련하여.. + Chat GPT (0) | 2023.04.02 |
[Next.js] localstorage is not defined (0) | 2023.03.25 |