모듈 참조
nest 에서는 내부 공급자 목록을 탐색하고, 동적으로 인스턴스화 하는 방법을 제공해 줍니다.
ModuleRef를 이용하여 클래스에 주입할 수 있습니다.
먼저 다음과 같은 파일 구조를 가지고 있다고 가정하겠습니다.
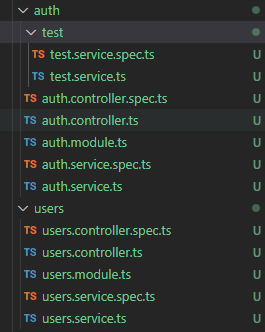
각 모듈의 코드를 살펴 보겠습니다.
auth.module.tsjavascriptimport { Module } from '@nestjs/common';
import { AuthController } from './auth.controller';
import { AuthService } from './auth.service';
import { TestService } from './test/test.service';
@Module({
imports: [],
controllers: [AuthController],
providers: [AuthService, TestService]
})
export class AuthModule {}
users.module.tsjavascriptimport { Module } from '@nestjs/common';
import { UsersController } from './users.controller';
import { UsersService } from './users.service';
@Module({
controllers: [UsersController],
providers: [UsersService]
})
export class UsersModule {}
보시는 거와 같이, user module은 service를 export 하지 않았고, auth module에서는 user module을 import 하지 않았습니다. 또한, .AuthModule에서는 내부 추가 서비스는 testService를 공급하고 있습니다.
다음은, auth service의 코드를 살펴보겠습니다.
auth.service.tsjavascriptimport { Injectable, OnModuleInit } from '@nestjs/common';
import { UsersService } from '../users/users.service';
import { ModuleRef } from '@nestjs/core';
import { TestService } from './test/test.service';
@Injectable()
export class AuthService implements OnModuleInit {
private userService: UsersService;
private testService: TestService;
constructor(private moduleRef: ModuleRef) {}
onModuleInit() {
this.userService = this.moduleRef.get(UsersService, {strict: false}) // 외부 참조
this.testService = this.moduleRef.get(TestService)
}
public test() {
return this.userService.test()
}
public test2() {
return this.testService.test()
}
}
위와 같이, moduleRef를 이용하여 내부 서비스인 test Service와 외부 서비스인 userService를 참조하였습니다.
각 결과값을 살펴보겠습니다.
auth.controller.tsjavascriptimport { Controller, Get } from '@nestjs/common';
import { AuthService } from './auth.service';
@Controller('auth')
export class AuthController {
constructor(private readonly authService: AuthService) {}
@Get('test')
public test() {
return this.authService.test()
}
@Get('test2')
public test2() {
return this.authService.test2()
}
}
그 전에 컨트롤러에서 대충 값을 찍을 수 있도록 처리해 줍니다.
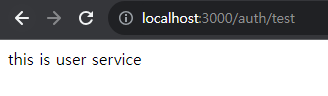
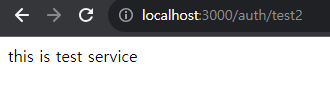
외부 서비스인 user 서비스와 내부 서비스인 test 서비스 모두 auth service에서 이용이 가능한 걸 확인할 수 있습니다.
하지만 외부 서비스인 경우 전반적으로 module 참조를 이용하는 편이 코드도 통일 되고 유지보수 하기에도 더 적합하다는 생각이 듭니다.
MockData 생성
모듈 참조의 다른 기능들을 보다가 적절한 사용 방법이 떠오르는게 해당 방안 정도만 생각나서 추가적으로 기술해 봅니다.
cats.factory.tsjavascriptimport { Injectable } from '@nestjs/common';
@Injectable()
export class CatsFactory {
private catList:Cat[] = [];
createCat(name: string, age: number): Cat {
this.catList.push({name, age})
return {
name,
age,
};
}
getCatList(): Cat[] {
return this.catList
}
}
interface Cat {
name: string;
age: number;
}
위와 같이 샘플 코드를 만들고,
moduleRef의 create를 이용하여 test시 사용하는 mockdata를 관리하는 용도로도 사용이 가능 할 것 같습니다.
cats.service.tsjavascriptimport { Injectable, OnModuleInit } from '@nestjs/common';
import { CatsFactory } from './cats.factory';
import { ModuleRef } from '@nestjs/core';
@Injectable()
export class CatsService implements OnModuleInit {
private catsFactory: CatsFactory;
constructor(private moduleRef: ModuleRef) {}
async onModuleInit() {
this.catsFactory = await this.moduleRef.create(CatsFactory);
// Test 시 mock data 생성?
this.catsFactory.createCat('hi',3)
this.catsFactory.createCat('hello',2)
console.dir(this.catsFactory)
}
}
'JAVASCRIPT > nest.js' 카테고리의 다른 글
[NestJS] NestJS + prisma CRUD (0) | 2024.06.11 |
---|---|
[nest.js] prisma schema 분리 (0) | 2024.06.04 |
[nest.js] nest.js + prisma - setting (0) | 2024.05.10 |
[Nest.js] Circular dependency (0) | 2023.10.02 |
모듈 참조
nest 에서는 내부 공급자 목록을 탐색하고, 동적으로 인스턴스화 하는 방법을 제공해 줍니다.
ModuleRef를 이용하여 클래스에 주입할 수 있습니다.
먼저 다음과 같은 파일 구조를 가지고 있다고 가정하겠습니다.
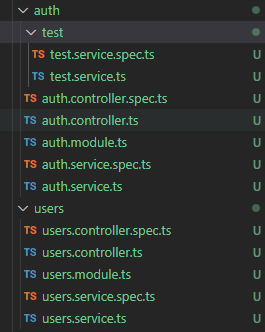
각 모듈의 코드를 살펴 보겠습니다.
auth.module.tsjavascriptimport { Module } from '@nestjs/common';
import { AuthController } from './auth.controller';
import { AuthService } from './auth.service';
import { TestService } from './test/test.service';
@Module({
imports: [],
controllers: [AuthController],
providers: [AuthService, TestService]
})
export class AuthModule {}
users.module.tsjavascriptimport { Module } from '@nestjs/common';
import { UsersController } from './users.controller';
import { UsersService } from './users.service';
@Module({
controllers: [UsersController],
providers: [UsersService]
})
export class UsersModule {}
보시는 거와 같이, user module은 service를 export 하지 않았고, auth module에서는 user module을 import 하지 않았습니다. 또한, .AuthModule에서는 내부 추가 서비스는 testService를 공급하고 있습니다.
다음은, auth service의 코드를 살펴보겠습니다.
auth.service.tsjavascriptimport { Injectable, OnModuleInit } from '@nestjs/common';
import { UsersService } from '../users/users.service';
import { ModuleRef } from '@nestjs/core';
import { TestService } from './test/test.service';
@Injectable()
export class AuthService implements OnModuleInit {
private userService: UsersService;
private testService: TestService;
constructor(private moduleRef: ModuleRef) {}
onModuleInit() {
this.userService = this.moduleRef.get(UsersService, {strict: false}) // 외부 참조
this.testService = this.moduleRef.get(TestService)
}
public test() {
return this.userService.test()
}
public test2() {
return this.testService.test()
}
}
위와 같이, moduleRef를 이용하여 내부 서비스인 test Service와 외부 서비스인 userService를 참조하였습니다.
각 결과값을 살펴보겠습니다.
auth.controller.tsjavascriptimport { Controller, Get } from '@nestjs/common';
import { AuthService } from './auth.service';
@Controller('auth')
export class AuthController {
constructor(private readonly authService: AuthService) {}
@Get('test')
public test() {
return this.authService.test()
}
@Get('test2')
public test2() {
return this.authService.test2()
}
}
그 전에 컨트롤러에서 대충 값을 찍을 수 있도록 처리해 줍니다.
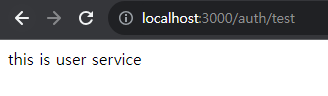
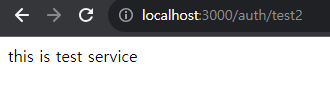
외부 서비스인 user 서비스와 내부 서비스인 test 서비스 모두 auth service에서 이용이 가능한 걸 확인할 수 있습니다.
하지만 외부 서비스인 경우 전반적으로 module 참조를 이용하는 편이 코드도 통일 되고 유지보수 하기에도 더 적합하다는 생각이 듭니다.
MockData 생성
모듈 참조의 다른 기능들을 보다가 적절한 사용 방법이 떠오르는게 해당 방안 정도만 생각나서 추가적으로 기술해 봅니다.
cats.factory.tsjavascriptimport { Injectable } from '@nestjs/common';
@Injectable()
export class CatsFactory {
private catList:Cat[] = [];
createCat(name: string, age: number): Cat {
this.catList.push({name, age})
return {
name,
age,
};
}
getCatList(): Cat[] {
return this.catList
}
}
interface Cat {
name: string;
age: number;
}
위와 같이 샘플 코드를 만들고,
moduleRef의 create를 이용하여 test시 사용하는 mockdata를 관리하는 용도로도 사용이 가능 할 것 같습니다.
cats.service.tsjavascriptimport { Injectable, OnModuleInit } from '@nestjs/common';
import { CatsFactory } from './cats.factory';
import { ModuleRef } from '@nestjs/core';
@Injectable()
export class CatsService implements OnModuleInit {
private catsFactory: CatsFactory;
constructor(private moduleRef: ModuleRef) {}
async onModuleInit() {
this.catsFactory = await this.moduleRef.create(CatsFactory);
// Test 시 mock data 생성?
this.catsFactory.createCat('hi',3)
this.catsFactory.createCat('hello',2)
console.dir(this.catsFactory)
}
}
'JAVASCRIPT > nest.js' 카테고리의 다른 글
[NestJS] NestJS + prisma CRUD (0) | 2024.06.11 |
---|---|
[nest.js] prisma schema 분리 (0) | 2024.06.04 |
[nest.js] nest.js + prisma - setting (0) | 2024.05.10 |
[Nest.js] Circular dependency (0) | 2023.10.02 |